Let’s discuss 10 basic C programs for interview success.
Program 1 : Addition of 2 numbers
#include<stdio.h>
#include<conio.h>
main()
{
int a;
int b;
int s;
clrscr();
a=56;
b=89;
s=a+b;
printf("%d",s);
}
The same C program can be written as below:
#include<stdio.h>
#include<conio.h>
main()
{
int a =56; b=89;
clrscr();
printf("%d +%d = %d",a,b,a+b);
}
The output of program depends on formatted printf()
The sample are tabulated as below:
printf() statement | Change in output |
printf(“%d”,s); | 145 |
printf(“sum=%d”,s); | sum=145 |
printf(“a+b=%d”,s); | a+b=145 |
printf(“%d +%d=%d”,a,b,s); | 56+89=145 |
printf(“%d + %d = %d”,a,b,a+b); | 56+89=145 |
printf(“Sum of %d and %d is %d”,a,b,s) | Sum of 56 and 89 is 145 |
Program Flow Chart
As per google , A flowchart is a type of diagram that represents a workflow or process.
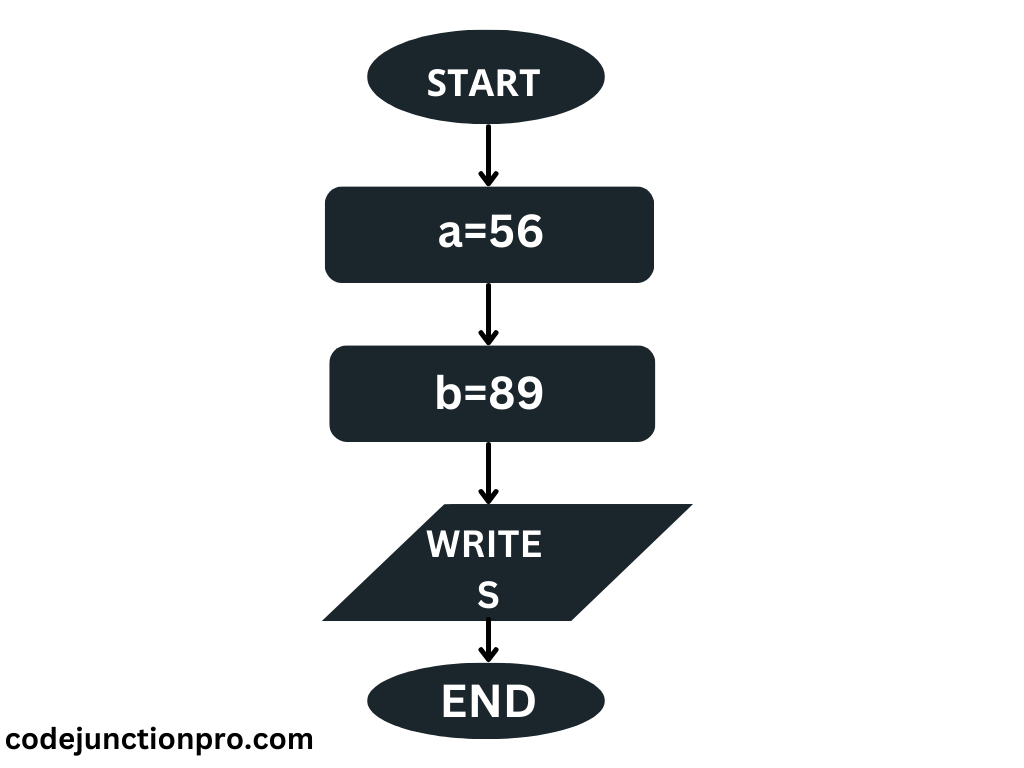
Program 2: Perform arithmetic operations on 2 integers
#include<stdio.h>
#include<conio.h>
main()
{
int a,b,s,d,p,r;
float q;
clrscr();
printf("Enter two numbers :");
scanf("%d%d",&a,&b);
s=a+b;
d=a-b;
p=a*b;
q=a/(float)b;
s=a+b;
d=a-b;
p=a*b;
q=a/(float)b;
r=a%b;
printf("a=%d and b=%d\n",a,b);
printf("\nSum = %d",s);
printf("\nDifference=%d",d);
printf("\nProduct = %d",p);
printf("\nQuotient = %f",q);
printf("\nReminder after interger division = %d",r);
Output:
Enter two numbers: 20 8
a = 20 and b =8
Sum = 28
Difference = 12
Product = 160
Quotient = 2.500000
Reminder after interger division = 4
Program 3: Calculate Area and Circumference of circle
#include<stdio.h>
#include<conio.h>
main()
{
int r;
float a,c;
clrscr();
printf("Enter radius of circle: ");
scanf("%d",&r);
a=3.14*r*r;
c=2*3.14*r;
printf("\nArea of circle is %f",a);
printf("\nCircumference of circle is %f",c);
Output:
Enter radius of circle: 10
Area of circle is 314.000000
Circumference of circle is 62.800000
Program 4: To Calculate Simple Interest (basic c programs for interview)
Simple Interest = PNR/100
Where,
P is principal amount
N is number of year (period)
R is rate of interest
#include<stdio.h>
#include<conio.h>
main()
{
int p,n
float r,I;
clrscr();
printf("Enter Principal Amount:");
scanf("%d",&p);
printf("Enter duration in years:");
scanf("%d",&n);
printf("Enter rate of interest:");
scanf("%f",&r);
I=p/100*r*n;
printf("\nSimple interest is %f",I);
}
Output:
Enter Principal Amount: 5000
Enter duration in years: 5
Enter rate of interest: 8.5
Simple interest is 2125.000000
Program 5: To convert temperature from degree Celsius to degree Fahrenheit (basic c programs for interview)
Fahrenheit = (9/5) * Celsius+32
i.e. F = 1.8 * C+32
Where,
F is Temperature in Fahrenheit
C is Temperature in Celsius
#include<stdio.h>
#include<conio.h>
main()
float f, c;
clrscr();
printf("Enter temperature in dergree Celsius:");
scanf("%f",&c);
f=1.8*c+32;
printf("\nTemperature in degree Fehrenheit is %.2f",f);
Output:
Enter temperature in degree Celsius: 32.5
Temperature in degree Fehrenheit is 90.50
Program 6: To swap(interchange) value of two variables by using a temporary variable (basic c programs for interview)
Suppose we input a=100 and b=200
Logic for swapping
Consider temporary variable t
Step 1: t = a => t = 100
Step 2: a = b => a = 200
Step 3: b= t => b = 100
After swapping a=200 and b=100
#include<stdio.h>
#include<conio.h>
main()
{
int a,b,t;
clrscr();
printf("Enter 2 numbers:");
scanf("%d%d",&a,&b);
t=a;
a=b;
b=t;
printf("\nAfter swapping\n");
printf("\na = %d",a);
printf("\nb =%d",b);
}
Program 7: To Swap(interchange) value of two variables without using third variable (basic c programs for interview)
Suppose we input a=100 and b=200
Logic for swapping
Step 1: a= a+b => a = 100 + 200=300
Step 2: b =a -b => b = 300-200=100
Step 3: a = a – b => a = 300-100=200
After swapping a=200 and b=100
#include<stdio.h>
#include<conio.h>
main()
{
int a,b,t;
clrscr();
printf("Enter 2 numbers:");
scanf("%d%d",&a,&b);
a=a+b;
b=a-b;
a=a-b;
printf("\nAfter swapping\n");
printf("\na =%d",a);
printf("\nb =%d",b);
}
Output:
Enter 2 numbers: 100 200
After swapping
a = 200
b = 100
Program 8: To input price in decimal form and print the output in paise
Suppose the price is 15.65
To convert into paise multiply it by 100
Logic for swapping
#include<stdio.h>
main()
{
float p;
int pp;
clrscr();
printf("\nEnter price in Rs.");
scanf("%f",&p);
pp=p*100;
printf("\nPrice in Paise %d",pp);
getch();
}
Output:
Enter price in Rs. 16.95
Price in Paise is 1695
Program 9: To input Roll number and Marks in 5 subjects out of 100 each. Calculate percentage and print it. (basic c programs for interview)
Note:
To Calculate percentage first calculate total marks by adding all marks. Then calculate percentage as
percentage = total * 100/500
or
percentage = total/5
#include<stdio.h>
main()
{
int roll_no;
int m1,m2,m3,m4,m5,total;
float per;
clrscr();
printf("Give Roll Number:");
scanf("%d",&roll_no);
printf("\nEnter marks in 5 subjects:");
scanf("%d%d%d%d%d",&m1,&m2,&m3,&m4,&m5);
total=m1+m2+m3+m4+m5;
per = total/5;
printf("\nRoll Number: %d",roll_no);
printf("\nMarks in subject 1: %d",m1);
printf("\nMarks in subject 2: %d",m2);
printf("\nMarks in subject 3: %d",m3);
printf("\nMarks in subject 4: %d",m4);
printf("\nMarks in subject 5: %d",m5);
printf("\nToatl Marks obtained out of 100 is %d",total);
printf("\nPercentage = %.2f",per);
getch();
}
Output:
Give Roll Number: 5
Enter marks in 5 subjects: 89 77 90 88 75
Roll Number: 5
Marks in Subject 1: 89
Marks in Subject 2: 77
Marks in Subject 3: 90
Marks in Subject 4: 88
Marks in Subject 5: 75
Total Marks obtained out of 100 is 419
Percentage = 83.80
Program 10: To calculate salary sheet of employee by inputting basic salary.
Consider the following computations.
DA (Dearness Allowance) = 21% of Basic
HRA (House Rent Allowance) = 15% of Basic
TA(Traveling Allowance) = 5% of Basic
Gross Salary = Basic + DA + HRA + TA
Insurance = Rs. 500
PF(Provident Fund) = 12% of Basic
Net Salary = Gross Salary – Insurance – PF
#include<stdio.h>
#include<conio.h>
main()
{
int b;
float d,h,t,gross,i,p,net;
clrscr();
printf("\nGive your Basic Salary:");
scanf("%d",&b);
d=b*0.21;
h=b*0.15;
t=b*0.05;
gross = b+d+h+t;
i=500;
p=b*0.12;
net=gross-(i+p);
printf("\nBasic Pay = Rs.%d",b);
printf("\nDA = Rs.%.2f",d);
printf("\nHRA = Rs.%.2f",h);
printf("\nTA = Rs.%.2f",t);
printf("\nGross Salary = Rs.%.2f",gross);
printf("\nInsurance = Rs.500");
printf("\nPF = Rs.%.2f",p);
printf("\n\nYour Net Salary is Rs. %.2f",net);
}
Output:
Give your Basic Salary: 12550
Basic Pay = Rs. 12550
DA = Rs. 2635.50
HRA = Rs. 1882.50
TA = Rs. 627.50
Gross Salary = Rs.17695.50
Insurance = Rs. 500
PF = Rs.1506.00
Your Net Salary is Rs.15689.50
One thought on “Basic C Programs for Interview Success: Key to Technical Excellence in 2023”